Mastering Mobile App Automation: A Roadmap to Readable and Maintainable Automated Tests
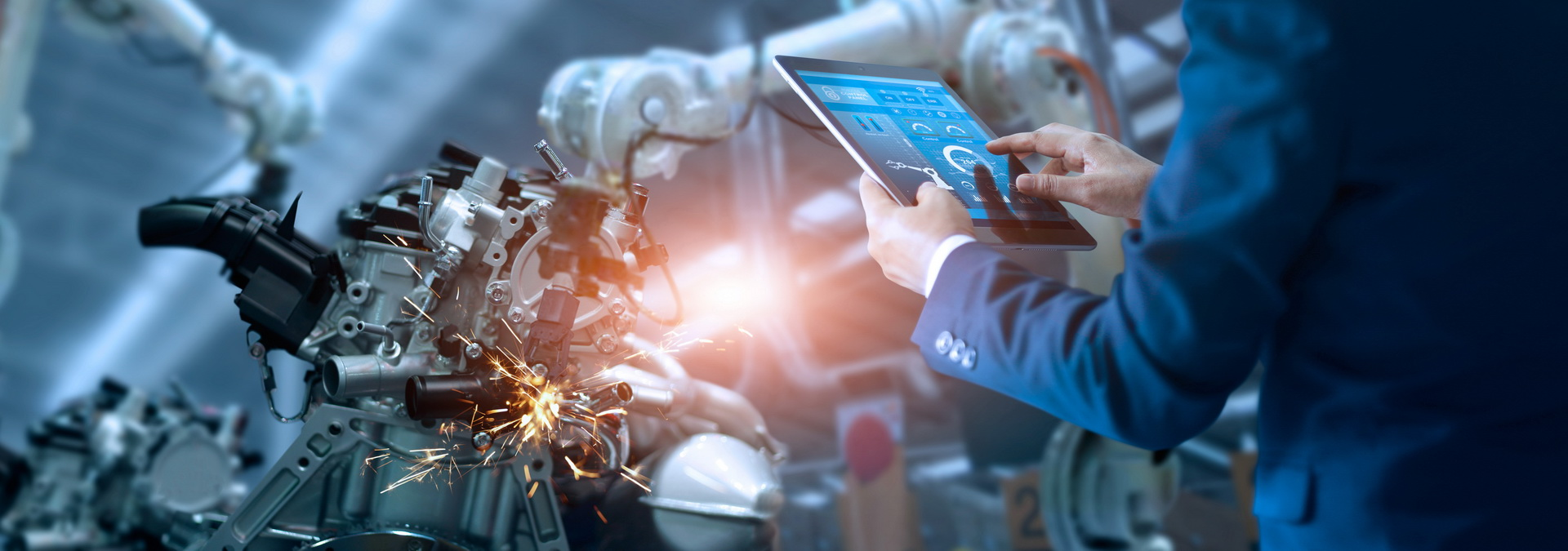
In the ever-expanding realm of mobile applications, ensuring their quality, functionality, and performance has become a paramount concern. Achieving these goals requires a robust and efficient automation framework, but one aspect often overlooked is the readability and maintainability of the automation tests. In this article, we will explore how adopting a readable and maintainable approach to mobile automation can not only enhance productivity but also elevate the overall quality of your software.
The Crucial Role of Readable and Maintainable Mobile Automation
The mobile app landscape is dynamic and competitive, with users expecting seamless experiences across various devices and platforms. To meet these expectations, thorough testing is essential, and this is where automation steps in. However, a well-structured and easily understandable automation framework can make a significant difference. We'll delve into the importance of readability and maintainability, how they influence testing outcomes, and the advantages they bring to the entire development process.
Prerequisites and Essential Tools for Embarking on Your Mobile Automation Journey
Before embarking on your journey into mobile automation, it's essential to lay a strong foundation by setting up the necessary tools and environment. Let's walk through the critical components you'll need to get started:
- Java: As the backbone of the majority of automation frameworks, Java is essential. Ensure you have the latest version of Java Development Kit (JDK) installed on your system. This powerful programming language will enable you to write robust and flexible automation tests.
- Maven: A build automation tool and project management tool combined, Maven helps you manage project dependencies, build lifecycles, and more. It simplifies the management of libraries, plugins, and external dependencies, making it a valuable asset for your mobile automation projects.
- Android Studio with Android SDK: If your mobile app testing includes Android devices, you'll need Android Studio along with the Android Software Development Kit (SDK). Android Studio is the official Integrated Development Environment (IDE) for Android app development, and it's indispensable for testing Android apps.
- Xcode: For iOS app testing, Xcode is a must. This integrated development environment by Apple allows you to build, test, and deploy iOS applications. Xcode includes the iOS Simulator, which is crucial for running your tests on iOS virtual devices.
- Appium 2.0: The latest version of Appium, 2.0, brings new features and improvements to the table. It's a powerful open-source tool for automating mobile applications on various platforms. It's cross-platform, which means you can write a single test script that can be used for both Android and iOS apps.
- Appium Android Driver: This driver is essential for automating interactions with Android devices. It allows you to interact with the Android operating system, perform gestures, and access elements on the screen, all critical for testing Android applications.
- Appium iOS Driver: Similar to the Android Driver, the iOS Driver is vital for iOS app testing. It enables you to interact with iOS devices, simulate gestures, and access elements on the iOS screen, ensuring comprehensive and accurate testing.
- IntelliJ IDEA: A robust Integrated Development Environment (IDE) is essential for writing, debugging, and running your automation tests. IntelliJ IDEA, with its powerful features and smooth integration with Maven, will be your partner in creating efficient and maintainable mobile automation tests.
By ensuring you have these tools set up correctly, you'll be ready to dive into the world of mobile automation with confidence. This solid foundation will empower you to harness the capabilities of these tools and create robust, readable, and maintainable automation tests, ultimately leading to higher-quality mobile applications.
The Power of the Page Object Design Pattern: Structuring for Reusability and Clarity
A cornerstone of creating maintainable and scalable automation tests, the Page Object Design Pattern has revolutionized the testing landscape. We'll explore in detail how this pattern enables you to encapsulate screen-specific logic, leading to more readable tests and reduced redundancy. By decoupling the test logic from the intricate details of the screens, you'll be better prepared to handle changes in the application with minimal effort, significantly extending the lifespan of your test suite.
Best Practices:
- Separation of Concerns: Keep the responsibilities of your screen classes and test classes distinct. A screen class should focus solely on housing locators and methods to interact with mobile elements. Avoid including assertions in the screen classes to maintain a clean separation of concerns.
- Method Grouping: Consider grouping related concrete methods within a screen class and creating higher-level methods based on their usage. For example, if your "LoginScreen.java" contains methods like "inputEmail()", "inputPassword()", and "clickOnLoginButton()", you can consolidate these into a private method called "login()". This practice enhances the readability of your code and makes it more intuitive, especially for business functions that involve multiple steps.
- Test Class Simplicity: Ensure that your test classes focus exclusively on test steps. Keep the test steps concise, clean, and easy to understand. Refrain from including locators or mobile elements directly within the test classes, as this can clutter the code and hinder maintainability.
- BaseScreen and BaseTest: Introduce a "BaseScreen.java" class that serves as the parent screen class. This class can hold common methods, such as wrappers for waiting and element interaction, promoting code reusability. Similarly, create a "BaseTest.java" as the parent test class to encapsulate shared setup and teardown procedures. By utilizing these base classes, you can streamline your code, improve reusability, and ensure consistency across your test suite.
Fluent Interface Design Pattern: Crafting Elegant and Expressive Test Code
Clear and concise test codes are a fundamental aspect of efficient communication among team members. The Fluent Interface Design Pattern empowers you to create tests that are not only precise but also remarkably easy to comprehend. We'll dive into the best practices for structuring your test codes using this pattern, enabling you to express your test scenarios in a natural and intuitive manner.
Best Practices:
- Consistent Screen Instance Return: When implementing the fluent interface pattern, ensure that your screen class methods consistently return the same screen instance. This practice enhances the fluidity and readability of your test code. By returning the same screen instance, you enable the chaining of methods in a natural and intuitive manner. It simplifies the structure of your test scripts and makes them more cohesive.
- Caution with Screen Instance Return: While returning a screen instance from methods is a powerful feature of the fluent interface, exercise caution not to overuse it. Only return the screen instance when it aligns with the intended use of the fluent pattern. Returning the screen instance when it's not necessary can lead to confusion and complicate the understanding of the code. It's essential to strike a balance between utilizing the fluent interface for clear method chaining and keeping the code concise and comprehensible.
Illustrative Instance of a Login Screen Class Leveraging The Best Practices
import io.appium.java_client.MobileBy;
import org.openqa.selenium.WebDriver;
public class LoginScreen extends BaseScreen {
public LoginScreen(WebDriver driver) {
super(driver);
}
public LoginScreen tapOnSignUpContainer() {
tap(MobileBy.AccessibilityId("button-sign-up-container"));
return this;
}
public void login(String emailAddress, String password) {
inputEmailAddress(emailAddress);
inputPassword(password);
tapOnLoginButton();
}
public void signUp(String emailAddress, String password, String confirmPassword) {
inputEmailAddress(emailAddress);
inputPassword(password);
inputConfirmPassword(confirmPassword);
tapOnSignUpButton();
}
private void inputEmailAddress(String emailAddress) {
inputText(MobileBy.AccessibilityId("input-email"), emailAddress);
}
private void inputPassword(String password) {
inputText(MobileBy.AccessibilityId("input-password"), password);
}
private void inputConfirmPassword(String confirmPassword) {
inputText(MobileBy.AccessibilityId("input-repeat-password"), confirmPassword);
}
private void tapOnLoginButton() {
tap(MobileBy.AccessibilityId("button-LOGIN"));
}
private void tapOnSignUpButton() {
tap(MobileBy.AccessibilityId("button-SIGN UP"));
}
}
Bringing It All Together: The Sample Project
Theory becomes truly valuable when it's put into practice. To reinforce your understanding, we'll guide you through a comprehensive sample mobile automation project that incorporates both the Page Object and Fluent Interface design patterns. This hands-on experience will provide you with the confidence to apply these concepts to your real-world testing scenarios, making your tests more efficient and less prone to breaking due to changes in the app.
GitHub Link: https://github.com/osandadeshan/appium-java-mobile-automation-demo
Conclusion: Elevating Your Mobile Automation Strategy
As we conclude our exploration, you'll have gained a comprehensive understanding of how a readable and maintainable approach to mobile automation can significantly enhance your testing strategy. Armed with the knowledge of design patterns and hands-on experience from the sample project, you'll be well-prepared to create efficient, effective, and long-lasting mobile automation tests. Bid farewell to convoluted, hard-to-maintain automation tests, and usher in a new era of mobile app testing that ensures quality, saves time, and empowers your development team. Investing in readability and maintainability is a smart investment in the future success of your mobile applications.
MagicPod is a no-code AI-driven test automation platform for testing mobile and web applications designed to speed up release cycles. Unlike traditional "record & playback" tools, MagicPod uses an AI self-healing mechanism. This means your test scripts are automatically updated when the application's UI changes, significantly reducing maintenance overhead and helping teams focus on development.